diff --git a/README.md b/README.md
index 35d6bf8c2..87f03067c 100644
--- a/README.md
+++ b/README.md
@@ -2,7 +2,6 @@
# LAB | JS Functions & Arrays
-
## Introduction
Manipulating arrays in code is a very common operation. Whether you're creating a total for a shopping cart, grabbing only the first names out of a list of people, or moving a piece on a chessboard, you're probably going to be modifying or manipulating an array in some way.
@@ -58,26 +57,28 @@ starter-code/
├── src
│ └── functions-and-arrays.js
├── tests
-│ └── FunctionsAndArraysSpec.js
+│ └── functions-and-arrays.spec.js
└─ SpecRunner.html
```
We will be working with the `functions-and-arrays.js` file inside of the `src` folder. In the `jasmine` folder you can find all of the files that compose Jasmine, that is already linked with the `SpecRunner.html` file.
-**Run tests**
+#### Run tests
Running automated tests with Jasmine is super easy. All you need to do is open the `SpecRunner.html` file in your browser. You will find something similar this:
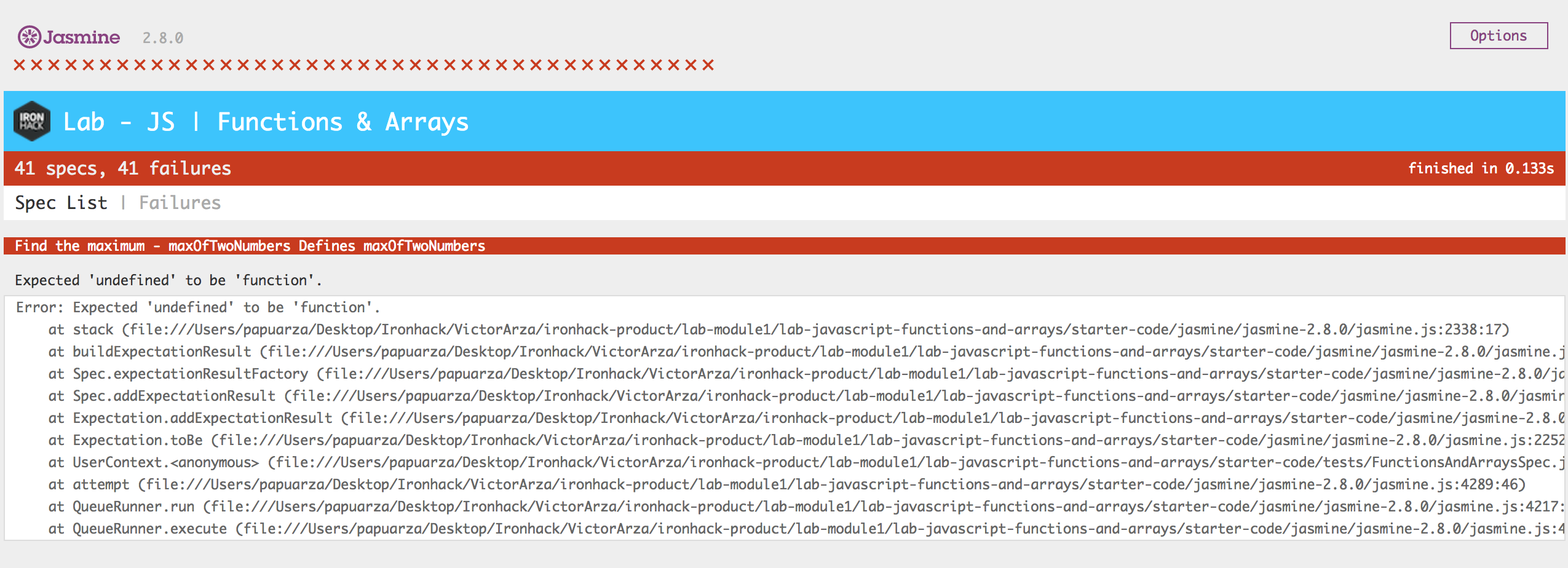
-**Pass the tests**
+#### Pass the tests
-You should write your code on the `src/functions-and-arrays.js` file. By following the instructions for each iteration, you should go every test and make sure it's _passing_.
+You should write your code on the `src/functions-and-arrays.js` file. While following the instructions for each iteration, you should check every test and make sure it's _passing_, before moving on.
Do not rush. You should take your time to carefully read every iteration, and you should address the _breaking_ tests as you progress through the exercise.
When coding with tests, it is super important that you carefully read and understand the errors you're getting, this way you'll know for sure what's expected from your code.
+Note that **you don't need to execute the functions yourself**, the tests are responsible for doing that. All you should do is declare them, make sure they deal with the parameters passed and that they return what is indicated on the iterations and in the test messages. For some iterations we provide you with a sample array, so that you can do some **manual** testing, if you wish.
+
## Deliverables
Write your JavaScript in the provided `src/functions-and-arrays.js` file.
@@ -86,11 +87,11 @@ Write your JavaScript in the provided `src/functions-and-arrays.js` file.
Define a function `maxOfTwoNumbers` that takes two numbers as arguments and returns the largest.
-## Iteration #2: Find longest word
+## Iteration #2: Find the longest word
Declare a function named `findLongestWord` that takes as an argument an array of words and returns the longest one. If there are 2 with the same length, it should return the first occurrence.
-**Starter Code**
+You can use the following array to test your solution:
```javascript
const words = ['mystery', 'brother', 'aviator', 'crocodile', 'pearl', 'orchard', 'crackpot'];
@@ -98,26 +99,42 @@ const words = ['mystery', 'brother', 'aviator', 'crocodile', 'pearl', 'orchard',
## Iteration #3: Calculate the sum
-Calculating a sum is as simple as iterating over an array and adding each of the elements together.
+Calculating a sum can be as simple as iterating over an array and adding each of the elements together.
-
+Declare a function named `sumArray` that takes an array of numbers as an argument, and returns the sum of all of the numbers in the array. Later in the course we'll learn how to do this by using the `reduce` array method, which will make your work significantly easier. For now, let's practice _"declarative"_ way adding values, using loops.
-Declare a function named `sumArray` that takes as an argument an array of numbers, and returns the sum of all of the numbers in the array. Later in the course we'll learn how to do this by using the `reduce` array method, which will make your work significantly easier. For now, let's practice _"manual"_ way using loops.
-
-**Starter Code**
+You can use the following array to test your solution:
```javascript
const numbers = [6, 12, 1, 18, 13, 16, 2, 1, 8, 10];
```
+### Bonus - Iteration #3.1: A generic `sum()` function
+
+**The goal: Learn how to refactor your code.** :muscle:
+
+In the iteration 3, you created a function that returns the sum of an array of numbers. But what if we wanted to know how much is the sum of the length of all of the words in an array? What if we wanted to add _boolean_ values to the mix? We wouldn't be able to use the same function as above, or better saying, we would have to _tweak_ it a little bit so that it can be reused no matter what is in the array that is passed as argument when function `sumArray()` is called.
+
+Here we're applying a concept we call **polymorphism**, that is, dealing with a functions' input independently of the types they're passed as.
+
+Let's create a new function `sum()` that calculates the sum for array filled with (_almost_) any type of data. Note that strings should have their length added to the total, and boolean values should be coerced into their corresponding numeric values. Check the tests for more details.
+
+You can use the following array to test your solution:
+
+```javascript
+const mixedArr = [6, 12, 'miami', 1, true, 'barca', '200', 'lisboa', 8, 10];
+
+// should return: 57
+```
+
## Iteration #4: Calculate the average
Calculating an average is an extremely common task. Let's practice it a bit.
-**Algorithm**
+**The logic behind this:**
-1. Find the sum as we did in the first exercise
-2. Take the sum from step 1, and divide it by the number of elements in the list.
+1. Find the sum as we did in the first exercise (or how about reusing that the _sumArray()_?)
+2. Take that sum and divide it by the number of elements in the list.
### Level 1: Array of numbers
@@ -150,6 +167,16 @@ const words = [
];
```
+### Bonus - Iteration #4.1: A generic `avg()` function
+
+Create function `avg(arr)` that receives any mixed array and calculates average. Consider as mixed array an array filled with numbers and/or strings and/or booleans. We're following a similar logic to the one applied on the bonus iteration 4.1 :wink:
+
+```javascript
+const mixedArr = [6, 12, 'miami', 1, true, 'barca', '200', 'lisboa', 8, 10];
+
+// should return: 5.7
+```
+
## Iteration #5: Unique arrays
Take the following array, remove the duplicates, and return a new array. You're more than likely going to want to check out the [`indexOf`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/indexOf) Array method.
@@ -217,11 +244,12 @@ const words = [
];
```
-## Iteration #8: Bonus
+## Bonus - Iteration #8: Product of adjacent numbers
-What is the greatest product of four adjacent numbers? We consider adjacent any four numbers that are next to each other in horizontal, vertical or diagonal.
+What is the greatest product of four adjacent numbers? We consider adjacent any four numbers that are next to each other horizontally or vertically.
For example, if we have a 5x5 Matrix like:
+
```bash
[ 1, 2, 3, 4, 5]
[ 1, 20, 3, 4, 5]
@@ -229,9 +257,10 @@ For example, if we have a 5x5 Matrix like:
[ 1, 20, 3, 4, 5]
[ 1, 4, 3, 4, 5]
```
+
The greatest product will be the `20`x`20`x`20`x`4` = `32000`;
-Declare a function named `greatestProduct` to find it in the 20×20 grid below!
+Declare a function named `greatestProduct(matrix)` to find it in the 20×20 grid below!
```javascript
const matrix = [
@@ -258,4 +287,8 @@ const matrix = [
];
```
+## Bonus - Iteration #8.1: Product of diagonals
+
+Following the logic you've used in iteration #8, declare a function called `greatestProductOfDiagonals(matrix)`. It takes a matrix as a parameter and returns the greatest product of any four values layed out diagonally, in either direction.
+
**Happy coding!** :heart:
diff --git a/starter-code/SpecRunner.html b/starter-code/SpecRunner.html
index ed617ecff..73c156ffd 100644
--- a/starter-code/SpecRunner.html
+++ b/starter-code/SpecRunner.html
@@ -1,24 +1,22 @@
-
-
- Jasmine Spec Runner v2.8.0
+
+
+ Jasmine Spec Runner v2.8.0
-
-
+
+
-
-
-
+
+
+
-
-
+
+
-
-
+
+
+
-
-
-
-
+
diff --git a/starter-code/src/functions-and-arrays.js b/starter-code/src/functions-and-arrays.js
index 467e533bb..720d3dcf5 100644
--- a/starter-code/src/functions-and-arrays.js
+++ b/starter-code/src/functions-and-arrays.js
@@ -12,18 +12,7 @@ const numbers = [6, 12, 1, 18, 13, 16, 2, 1, 8, 10];
const numbersAvg = [2, 6, 9, 10, 7, 4, 1, 9];
// Level 2: Array of strings
-const wordsArr = [
- 'seat',
- 'correspond',
- 'linen',
- 'motif',
- 'hole',
- 'smell',
- 'smart',
- 'chaos',
- 'fuel',
- 'palace'
-];
+const wordsArr = ['seat', 'correspond', 'linen', 'motif', 'hole', 'smell', 'smart', 'chaos', 'fuel', 'palace'];
// Iteration #5: Unique arrays
const wordsUnique = [
@@ -41,16 +30,7 @@ const wordsUnique = [
];
// Iteration #6: Find elements
-const wordsFind = [
- 'machine',
- 'subset',
- 'trouble',
- 'starting',
- 'matter',
- 'eating',
- 'truth',
- 'disobedience'
-];
+const wordsFind = ['machine', 'subset', 'trouble', 'starting', 'matter', 'eating', 'truth', 'disobedience'];
// Iteration #7: Count repetition
const wordsCount = [
diff --git a/starter-code/tests/FunctionsAndArraysSpec.js b/starter-code/tests/FunctionsAndArraysSpec.js
deleted file mode 100644
index 05e177082..000000000
--- a/starter-code/tests/FunctionsAndArraysSpec.js
+++ /dev/null
@@ -1,298 +0,0 @@
-function shuffle(currentArray) {
- const array = currentArray.map(arr => arr.slice());
- let counter = array.length;
-
- while (counter > 0) {
- let index = Math.floor(Math.random() * counter);
- counter--;
- let temp = array[counter];
- array[counter] = array[index];
- array[index] = temp;
- }
- return array;
-}
-
-describe('Find the maximum - maxOfTwoNumbers', () => {
- it('Defines maxOfTwoNumbers', () => {
- expect(typeof maxOfTwoNumbers).toBe('function');
- });
-
- it('First parameter larger', () => {
- expect(maxOfTwoNumbers(2, 1)).toBe(2);
- });
-
- it('Second parameter larger', () => {
- expect(maxOfTwoNumbers(1, 3)).toBe(3);
- });
-
- it('First and Second parameter equal', () => {
- expect(maxOfTwoNumbers(4, 4)).toBe(4);
- });
-});
-
-describe('Finding Longest Word - findLongestWord', () => {
- it('Defines findLongestWord', function() {
- expect(typeof findLongestWord).toBe('function');
- });
-
- it('returns null when called with an empty array', () => {
- expect(findLongestWord([])).toBe(null);
- });
-
- it('returns the word when called with a single-word array', () => {
- expect(findLongestWord(['foo'])).toBe('foo');
- });
-
- it('returns the first occurrence word when longest have multiple occurrences ', () => {
- expect(findLongestWord(['foo', 'bar'])).toBe('foo');
- expect(findLongestWord(['bar', 'foo'])).toBe('bar');
- });
-
- it('returns the longest occurrence when it has multiple words', () => {
- let words = ['a', 'zab', '12abc', '$$abcd', 'abcde', 'ironhack'];
- for (let i = 0; i < 10; i++) {
- words = shuffle(words);
- expect(findLongestWord(words)).toBe('ironhack');
- }
- });
-});
-
-describe('Calculating a Sum - sumArray', () => {
- it('Defines sumArray', () => {
- expect(typeof sumArray).toBe('function');
- });
-
- it('returns zero with an empty array', () => {
- expect(sumArray([])).toBe(0);
- });
-
- it('returns the sum with one number array', () => {
- expect(sumArray([4])).toBe(4);
- });
-
- it('returns zero if all elements are zero', () => {
- expect(sumArray([0, 0, 0, 0, 0])).toBe(0);
- });
-
- it('returns the sum', () => {
- expect(sumArray([10, 5, 4, 32, 8])).toBe(59);
- });
-});
-
-describe('Calculating the Average - averageNumbers', () => {
- it('Defines averageNumbers', () => {
- expect(typeof averageNumbers).toBe('function');
- });
-
- it('returns null with an empty array', () => {
- expect(averageNumbers([])).toBe(null);
- });
-
- it('returns the average of a unique element array', () => {
- expect(averageNumbers([9])).toBe(9);
- });
-
- it('returns the average even with negative values', () => {
- expect(averageNumbers([9, -3, -4, 6])).toBe(2);
- });
-
- it('returns the average of the array', () => {
- expect(averageNumbers([9, 10, 82, 92, 32, 102, 58])).toBe(55);
- });
-});
-
-describe('Calculating the Average - averageWordLength', () => {
- it('Defines averageWordLength', () => {
- expect(typeof averageWordLength).toBe('function');
- });
-
- it('returns null with an empty array', () => {
- expect(averageWordLength([])).toBe(null);
- });
-
- it('returns the average of a unique element array', () => {
- expect(averageWordLength(['ironhack'])).toBe(8);
- });
-
- it('returns the average of a the array', () => {
- expect(
- averageWordLength([
- 'Ironhack',
- 'Madrid',
- 'Barcelona',
- 'Paris',
- 'Miami',
- 'Mexico',
- 'Berlin',
- 'Programmers'
- ])
- ).toBe(7);
- });
-});
-
-describe('Unique Arrays - uniquifyArray', () => {
- it('Defines uniquifyArray', () => {
- expect(typeof uniquifyArray).toBe('function');
- });
-
- it('returns [] with an empty array', () => {
- expect(uniquifyArray([])).toEqual([]);
- });
-
- it('returns the correct array when having an array of the same element', () => {
- expect(uniquifyArray(['Ironhack', 'Ironhack', 'Ironhack'])).toEqual([
- 'Ironhack'
- ]);
- });
-
- it('returns the same array when no element is repeated', () => {
- expect(uniquifyArray(['Cat', 'Dog', 'Cow'])).toEqual(['Cat', 'Dog', 'Cow']);
- });
-
- it('returns the uniquified array', () => {
- expect(
- uniquifyArray([
- 'iPhone',
- 'Samsung',
- 'Android',
- 'iOS',
- 'iPhone',
- 'Samsung',
- 'Nokia',
- 'Blackberry',
- 'Android'
- ])
- ).toEqual(['iPhone', 'Samsung', 'Android', 'iOS', 'Nokia', 'Blackberry']);
- });
-});
-
-describe('Finding Elements - doesWordExist', () => {
- it('Defines doesWordExist', () => {
- expect(typeof doesWordExist).toBe('function');
- });
-
- it('returns false with an empty array', () => {
- expect(doesWordExist([])).toBe(false);
- });
-
- it('returns true if the word we are looking is the only one on the array', () => {
- expect(doesWordExist(['machine'], 'machine')).toBe(true);
- });
-
- it('returns false if the word we are looking is not in the array', () => {
- expect(
- doesWordExist(
- ['machine', 'poison', 'eat', 'apple', 'horse'],
- 'ratatouille'
- )
- ).toBe(false);
- });
-
- it('returns true if the word we are looking is in the array', () => {
- expect(
- doesWordExist(
- ['pizza', 'sandwich', 'snack', 'soda', 'book', 'computer'],
- 'book'
- )
- ).toBe(true);
- });
-});
-
-describe('Counting Repetition - howManyTimes', () => {
- it('Defines howManyTimes', () => {
- expect(typeof howManyTimes).toBe('function');
- });
-
- it('returns 0 with an empty array', () => {
- expect(howManyTimes([])).toBe(0);
- });
-
- it('returns one when the word appears only one time on the array', () => {
- expect(howManyTimes(['basketball', 'football', 'tennis'], 'tennis')).toBe(
- 1
- );
- });
-
- it('returns zero when the word does not appears on the array', () => {
- expect(howManyTimes(['basketball', 'football', 'tennis'], 'rugby')).toBe(0);
- });
-
- it('returns five when the word appears 5 times on the array', () => {
- expect(
- howManyTimes(
- [
- 'basketball',
- 'football',
- 'tennis',
- 'rugby',
- 'rugby',
- 'ping pong',
- 'rugby',
- 'basketball',
- 'rugby',
- 'handball',
- 'rugby'
- ],
- 'rugby'
- )
- ).toBe(5);
- });
-});
-
-describe('Bonus Quest - greatestProduct', () => {
- it('Defines greatestProduct', () => {
- expect(typeof greatestProduct).toBe('function');
- });
-
- it('Return 1 when all the numbers of the arrays are 1', () => {
- let matrix = [
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
- [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
- ];
- expect(greatestProduct(matrix)).toBe(1);
- });
-
- it('Return 16 when all the numbers of the arrays are 2', () => {
- let matrix = [
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
- [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2]
- ];
- expect(greatestProduct(matrix)).toBe(16);
- });
-});
diff --git a/starter-code/tests/functions-and-arrays.spec.js b/starter-code/tests/functions-and-arrays.spec.js
new file mode 100644
index 000000000..6de4eaed4
--- /dev/null
+++ b/starter-code/tests/functions-and-arrays.spec.js
@@ -0,0 +1,365 @@
+function shuffle(currentArray) {
+ const array = currentArray.map(arr => arr.slice());
+ let counter = array.length;
+
+ while (counter > 0) {
+ let index = Math.floor(Math.random() * counter);
+ counter--;
+ let temp = array[counter];
+ array[counter] = array[index];
+ array[index] = temp;
+ }
+ return array;
+}
+
+describe('Find the maximum', () => {
+ it('should create a function named maxOfTwoNumbers', () => {
+ expect(typeof maxOfTwoNumbers).toBe('function');
+ });
+
+ it('should return greater of two arguments - if the first argument greater', () => {
+ expect(maxOfTwoNumbers(2, 1)).toBe(2);
+ expect(maxOfTwoNumbers(5, -7)).toBe(5);
+ });
+
+ it('should return greater of two arguments - if the second argument greater', () => {
+ expect(maxOfTwoNumbers(1, 3)).toBe(3);
+ expect(maxOfTwoNumbers(-5, 3)).toBe(3);
+ });
+
+ it('should return either arguments - if both arguments are equal', () => {
+ expect(maxOfTwoNumbers(4, 4)).toBe(4);
+ });
+});
+
+describe('Find the longest word', () => {
+ it('should create a function named findLongestWord', () => {
+ expect(typeof findLongestWord).toBe('function');
+ });
+
+ it('should return null when called with an empty array', () => {
+ expect(findLongestWord([])).toBe(null);
+ });
+
+ it('should return the word when called with a single-word array', () => {
+ expect(findLongestWord(['foo'])).toBe('foo');
+ });
+
+ it('should return the first occurrence of the word when longest have multiple occurrences ', () => {
+ expect(findLongestWord(['foo', 'bar'])).toBe('foo');
+ expect(findLongestWord(['bar', 'foo'])).toBe('bar');
+ });
+
+ it('should return the longest occurrence when it has multiple words', () => {
+ let words = ['a', 'zab', '12abc', '$$abcd', 'abcde', 'ironhack'];
+ for (let i = 0; i < 10; i++) {
+ words = shuffle(words);
+ expect(findLongestWord(words)).toBe('ironhack');
+ }
+ });
+});
+
+describe('Calculate the sum of array of numbers', () => {
+ it('should create a function named sumNumbers', () => {
+ expect(typeof sumNumbers).toBe('function');
+ });
+
+ it('should return zero if receives an empty array when called', () => {
+ expect(sumNumbers([])).toBe(0);
+ });
+
+ it('should return the sum with one number array', () => {
+ expect(sumNumbers([4])).toBe(4);
+ });
+
+ it('should return zero if all elements are zero', () => {
+ expect(sumNumbers([0, 0, 0, 0, 0])).toBe(0);
+ });
+
+ it('should return the sum when passed array of numbers', () => {
+ expect(sumNumbers([10, 5, 4, 32, 8])).toBe(59);
+ });
+});
+
+describe('Bonus: Calculate the sum', () => {
+ it('should create a function named sum', () => {
+ expect(typeof sum).toBe('function');
+ });
+
+ it('should return zero if receives an empty array when called', () => {
+ expect(sum([])).toBe(0);
+ });
+
+ it('should return the sum with one number array', () => {
+ expect(sum([4])).toBe(4);
+ });
+
+ it('should return zero if all elements are zero', () => {
+ expect(sum([0, 0, 0, 0, 0])).toBe(0);
+ });
+
+ it('should return the sum when passed array of numbers', () => {
+ expect(sum([10, 5, 4, 32, 8])).toBe(59);
+ });
+
+ it('should return the sum when passed array of strings', () => {
+ expect(sum(['ana', 'marco', 'nicolas', 'tania', 'ptwd'])).toBe(24);
+ });
+
+ it('should return the sum when passed array of mixed strings and numbers - ', () => {
+ expect(sum([6, 12, 'miami', 1, 'barca', '200', 'lisboa', 8, 10])).toBe(56);
+ });
+ it('should return the sum when passed array of mixed strings, numbers and booleans - ', () => {
+ // false is counted as 0
+ expect(sum([6, 12, 'miami', 1, 'barca', '200', 'lisboa', 8, false])).toBe(46);
+ // true is counted as 1
+ expect(sum([6, 12, 'miami', 1, 'barca', '200', 'lisboa', 8, true])).toBe(47);
+ });
+ it('should throw an error when unsupported data type (object or array) present in the array', () => {
+ // const arr = [6, 12, "miami", 1, "barca", "200", "lisboa", 8, [], {}];
+
+ expect(() => sum([6, 12, 'miami', 1, 'barca', '200', 'lisboa', 8, [], {}])).toThrow(
+ new Error("Unsupported data type sir or ma'am")
+ );
+ });
+});
+
+describe('Calculate the average of an array of numbers', () => {
+ it('should create a function named averageNumbers', () => {
+ expect(typeof averageNumbers).toBe('function');
+ });
+
+ it('should return null if receives an empty array when called', () => {
+ // should it return null or zero?
+ expect(averageNumbers([])).toBe(null);
+ });
+
+ // do we need this?
+ // it("should return the average of a one-element array", () => {
+ // expect(averageNumbers([9])).toBe(9);
+ // });
+
+ // do we need this?
+ // it("should return the average even with negative values", () => {
+ // expect(averageNumbers([9, -3, -4, 6])).toBe(2);
+ // });
+
+ it('should return the average of the array', () => {
+ expect(averageNumbers([9, 10, 82, 92, 32, 102, 58])).toBe(55);
+ });
+});
+
+describe('Calculate the average of an array of strings', () => {
+ it('should create a function named averageWordLength', () => {
+ expect(typeof averageWordLength).toBe('function');
+ });
+
+ it('should return null if receives an empty array when called', () => {
+ // should it return null or zero?
+ expect(averageWordLength([])).toBe(null);
+ });
+
+ // do we need this?
+ // it("should return the average of a one-element array", () => {
+ // expect(averageWordLength(["ironhack"])).toBe(8);
+ // });
+
+ it('should return the average of a the array', () => {
+ expect(
+ averageWordLength([
+ 'Ironhack',
+ 'Madrid',
+ 'Barcelona',
+ 'Paris',
+ 'Miami',
+ 'Mexico',
+ 'Berlin',
+ 'Programmers'
+ ])
+ ).toBe(7);
+ });
+});
+
+describe('Bonus: Calculate the average of a mixed elements array', () => {
+ it('should create a function named avg', () => {
+ expect(typeof avg).toBe('function');
+ });
+
+ it('should return null if receives an empty array when called', () => {
+ // should it return null or zero?
+ expect(avg([])).toBe(null);
+ });
+
+ it('should return the average of the array', () => {
+ // false is counted as 0
+ expect(avg([6, 12, 'miami', 1, 'barca', '200', 'lisboa', 8, false])).toBe(5.11);
+ // true is counted as 1
+ expect(avg([6, 12, 'miami', 1, 'barca', '200', 'lisboa', 8, true])).toBe(5.22);
+ });
+});
+
+describe('Unique array', () => {
+ it('should create a function named uniquifyArray', () => {
+ expect(typeof uniquifyArray).toBe('function');
+ });
+
+ it('should return null if receives an empty array when called', () => {
+ expect(uniquifyArray([])).toEqual(null);
+ });
+
+ // do we need this?
+ // it("should return the correct uniqified array when an array of the same elements passed as argument", () => {
+ // expect(uniquifyArray(["Ironhack", "Ironhack", "Ironhack"])).toEqual([
+ // "Ironhack"
+ // ]);
+ // });
+
+ // do we need this?
+ // it("should return the same array when no element is repeated", () => {
+ // expect(uniquifyArray(["Cat", "Dog", "Cow"])).toEqual(["Cat", "Dog", "Cow"]);
+ // });
+
+ it('should return the uniquified array', () => {
+ expect(
+ uniquifyArray([
+ 'iPhone',
+ 'Samsung',
+ 'Android',
+ 'iOS',
+ 'iPhone',
+ 'Samsung',
+ 'Nokia',
+ 'Blackberry',
+ 'Android'
+ ])
+ ).toEqual(['iPhone', 'Samsung', 'Android', 'iOS', 'Nokia', 'Blackberry']);
+ });
+});
+
+describe('Find elements', () => {
+ it('should create a function named doesWordExist', () => {
+ expect(typeof doesWordExist).toBe('function');
+ });
+
+ it('should return null if receives an empty array when called', () => {
+ expect(doesWordExist([])).toBe(null);
+ });
+
+ // do we need this test?
+ // it("should return true if the word we are looking for is the only one in the array", () => {
+ // expect(doesWordExist(["machine"], "machine")).toBe(true);
+ // });
+
+ // do we need this test?
+ // it("should return false if the word we are looking for is not in the array", () => {
+ // expect(
+ // doesWordExist(
+ // ["machine", "poison", "eat", "apple", "horse"],
+ // "ratatouille"
+ // )
+ // ).toBe(false);
+ // });
+
+ it('should return true if the word we are looking for is in the array', () => {
+ expect(doesWordExist(['pizza', 'sandwich', 'snack', 'soda', 'book', 'computer'], 'book')).toBe(
+ true
+ );
+ });
+});
+
+describe('Count repetition', () => {
+ it('should create a function named howManyTimes', () => {
+ expect(typeof howManyTimes).toBe('function');
+ });
+
+ it('should return 0 (zero) if receives an empty array when called', () => {
+ expect(howManyTimes([])).toBe(0);
+ });
+
+ it('should return 1 (one) when the word appears only one time in the array', () => {
+ expect(howManyTimes(['basketball', 'football', 'tennis'], 'tennis')).toBe(1);
+ });
+
+ it("should return 0 (zero) when the word doesn't appear in the array", () => {
+ expect(howManyTimes(['basketball', 'football', 'tennis'], 'rugby')).toBe(0);
+ });
+
+ it('should return 5 (five) when the word appears 5 times in the array', () => {
+ expect(
+ howManyTimes(
+ [
+ 'basketball',
+ 'football',
+ 'tennis',
+ 'rugby',
+ 'rugby',
+ 'ping pong',
+ 'rugby',
+ 'basketball',
+ 'rugby',
+ 'handball',
+ 'rugby'
+ ],
+ 'rugby'
+ )
+ ).toBe(5);
+ });
+});
+
+describe('Bonus Quest - greatestProduct', () => {
+ it('should create a function named greatestProduct', () => {
+ expect(typeof greatestProduct).toBe('function');
+ });
+
+ it('should return 1 (one) when all numbers of the arrays are 1', () => {
+ let matrix = [
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
+ [1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
+ ];
+ expect(greatestProduct(matrix)).toBe(1);
+ });
+
+ it('should return 16 when all the numbers of the arrays are 2', () => {
+ let matrix = [
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
+ [2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2]
+ ];
+ expect(greatestProduct(matrix)).toBe(16);
+ });
+});